Magic IndexedDB - Blazor Introduction
Magic IndexedDB - Blazor Introduction
Author: Lance Wright II
Published: June 01, 2025
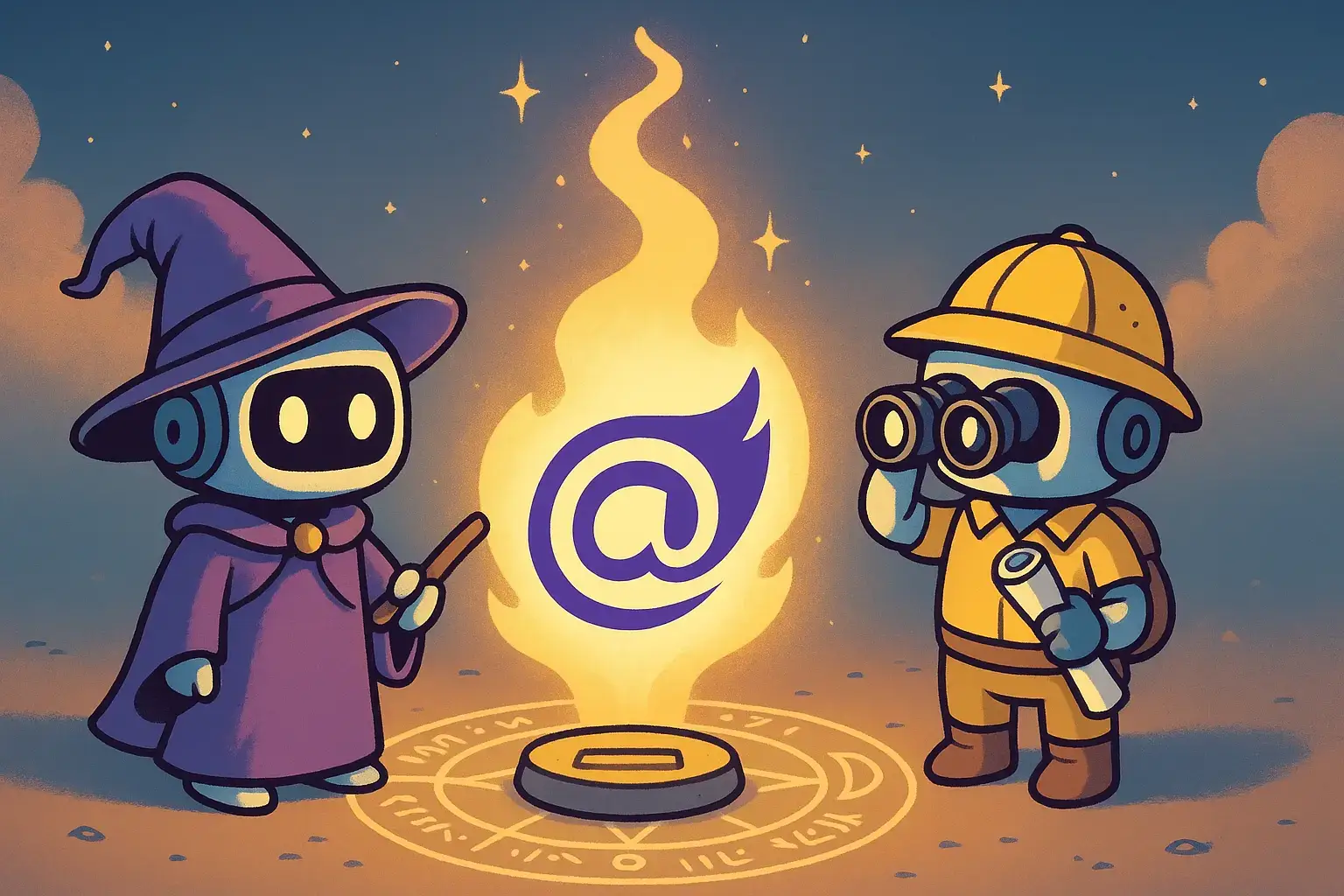
This is the introduction to utilizing Magic IndexedDB in Blazor.
Introduction to LINQ to IndexedDB in Blazor
1. Getting Started
Before we dive into queries, let's first set up Magic IndexedDB in your Blazor pages.
🔹 Add Magic IndexedDB to `_Imports.razor`
To avoid writing @using Magic.IndexedDb on every page, add it to your `_Imports.razor`:
@using Magic.IndexedDb
🔹 Inject Magic IndexedDB Into Your Pages
In any Blazor page or component where you want to use Magic IndexedDB, inject the service at the top:
@inject IMagicIndexedDb _MagicDb
Boom! You're plugged in and ready to go! ๐
2. Initializing a Query
Now that you've injected _MagicDb, you can start querying IndexedDB! There are four ways to initialize a query:
✅ 1. Default Database Query
IMagicQuery<Person> personQuery = await _MagicDb.Query<Person>();
Targets the default database associated with Person
.
If your Person
table is linked to a single database, this is the best way to query it.
✅ 2. Querying an Assigned Database (Strongly Typed)
IMagicQuery<Person> employeeDbQuery = await _MagicDb.Query<Person>(x => x.Databases.Client);
Explicitly specifies which database to query.
Person
is configured to exist in the Client
database, so this is a safe and recommended approach.
⚠️ 3. Querying an Unassigned Database (Not Recommended)
IMagicQuery<Person> animalDbQuery = await _MagicDb.Query<Person>(IndexDbContext.Animal);
Person
does not belong to the Animal
database.
Magic IndexedDB allows this for flexibility, but it's discouraged.
Why? It bypasses safety checks and may require manual migrations.
🚨 4. Full Override Query (Strongly Discouraged)
IMagicQuery<Person> unassignedDbQuery = await _MagicDb.QueryOverride<Person>("DbName", "SchemaName");
Completely overrides database and schema restrictions.
Magic IndexedDB cannot protect you if you use this.
DO NOT DO THIS unless you absolutely know what you're doing.
โ Avoid unassigned queries and full overrides unless necessary.
- They break the built-in migration system.
- They require manual fixes when schema updates occur.
- You lose all built-in protection mechanisms.
Which One Should You Use?
Query Type | Safety Level | When to Use? |
---|---|---|
await _MagicDb.Query<Person>(); |
โ Safe | When Person has one default database. |
await _MagicDb.Query<Person>(x => x.Databases.Client); |
โ Safe | When Person has multiple valid databases. |
await _MagicDb.Query<Person>(IndexDbContext.Animal); |
โ ๏ธ Risky | When Person is not part of the specified database. |
await _MagicDb.QueryOverride<Person>("DbName", "SchemaName"); |
๐จ Dangerous | Only for advanced niche use cases. |
3. Basic CRUD Operations
Now that you know how to query, let's look at basic IndexedDB operations.
🔹 Adding Data
await personQuery.AddAsync(new Person { Name = "John Doe", Age = 30 });
🔹 Adding Multiple Records (Batch Insert)
List<Person> persons = new List<Person>
{
new Person { Name = "Alice", Age = 25 },
new Person { Name = "Bob", Age = 28 }
};
await personQuery.AddRangeAsync(persons);
🔹 Updating Data
var person = new Person { Name = "John Doe", Age = 31 };
await personQuery.UpdateAsync(person);
🔹 Updating Multiple Records
await personQuery.UpdateRangeAsync(persons);
🔹 Deleting Data
await personQuery.DeleteAsync(person);
🔹 Deleting Multiple Records
await personQuery.DeleteRangeAsync(persons);
🚀 Bulk Clearing a Table
await personQuery.ClearTable();
Deletes all data inside the table instantly.
Use with caution! ๐ฅ
Using LINQ to IndexedDB – Query Syntax & Best Practices
1. Supported Query Operations
Magic IndexedDB fully supports LINQ-style queries, enabling powerful data retrieval and filtering while enforcing best practices to ensure optimal IndexedDB performance.
There are two primary query operations in Magic IndexedDB:
1๏ธโฃ .Where(x => YourPredicate)
โ Optimized for Indexed Queries
2๏ธโฃ .Cursor(x => YourPredicate)
โ Meta-data Driven Cursor Queries
Before we dive in, there's something critical you must know:
🚨 IndexedDB Has a Backwards Take/Skip Order!
In every language and database system, Skip()
is always before Take()
.
โ
Example in SQL:
SELECT * FROM People ORDER BY Age LIMIT 10 OFFSET 5;
Here, OFFSET 5 (skip) happens before LIMIT 10 (take).
๐จ In IndexedDB, this is reversed.
If you call Skip(5).Take(10)
, only Skip(5)
will apply
If you call Take(10).Skip(5)
, it works correctly
๐ฅ Magic IndexedDB handles this for youโbut you must always write Take()
before Skip()
to avoid unexpected behavior.
This is also an enforced behavior by the interfaces to protect you. As the interfaces on the query won't allow you to append any Take
or TakeLast
after a Skip
.
2. Using `.Where()` for Optimized Queries
The .Where()
method translates LINQ expressions into the most efficient IndexedDB queries possible.
Multiple .Where()
statements can be chained together.
✅ Basic `.Where()` Query
await personQuery.Where(x => x.Age > 30)
.Where(x => x.TestInt == 9 || x.Name.StartsWith("C", StringComparison.OrdinalIgnoreCase))
.ToListAsync();
Multiple .Where()
clauses are supported.
Deferred execution works identically to LINQ to SQL.
Queries execute with ToListAsync()
or AsAsyncEnumerable()
.
🔹 Streaming Results with `AsAsyncEnumerable()`
await foreach (var person in personQuery.Where(x => x.Age > 30).AsAsyncEnumerable())
{
Console.WriteLine($"Name: {person.Name}");
}
Yields results as they come in.
Reduces memory usage when processing large datasets.
Enforces unique ID consistency across results.
๐ Magic IndexedDBโs custom-built interop layer enables real-time yielding.
However, note that yielding incurs additional serialization costs, making bulk returns faster.
3. Query Execution Model – Understanding Indexed vs. Cursor Queries
Magic IndexedDB automatically categorizes queries into one of three optimized execution types:
✅ Indexed Queries
Fastest queries.
Utilize IndexedDBโs built-in indexing.
Example:
await personQuery.Where(x => x.Name == "Alice").ToListAsync();
Since Name
has an index, it fires an Indexed Query.
⚡ Combined Indexed Queries
Utilizes multiple indexes together for efficient searching.
More powerful than standard IndexedDB queries.
Example:
await personQuery.Where(x => x.Age > 30 && x.TestInt == 9).ToListAsync();
If both Age
and TestInt
are indexed, this is an optimized combined indexed query.
🛑 Cursor-Based Queries (Meta-Data Driven)
Slower, but allows for more complex filtering.
Keeps only meta-data in memory until the exact desired result is known.
Example:
await personQuery.Where(x => x.Name.Contains("bo", StringComparison.OrdinalIgnoreCase)).ToListAsync();
Since Contains()
is not natively indexed, a cursor query fires instead.
๐น The more your query relies on cursors, the slower it will be.
To optimize performance, ensure your tables have proper indexes!
4. Query Interface Enforcements & Best Practices
To prevent you from accidentally breaking IndexedDB optimizations, Magic IndexedDB enforces best practices using interfaces that restrict unsafe query combinations.
Query Flow – How Queries Are Structured
public interface IMagicQuery<T> : IMagicExecute<T> where T : class
This enforces safe LINQ structures.
Query Staging – Ensuring IndexedDB-Compatible Queries
public interface IMagicQueryStaging<T> : IMagicExecute<T> where T : class
{
IMagicQueryStaging<T> Where(Expression<Func<T, bool>> predicate);
IMagicQueryPaginationTake<T> Take(int amount);
IMagicQueryFinal<T> Skip(int amount);
IMagicQueryOrderable<T> OrderBy(Expression<Func<T, object>> predicate);
}
Once you call .Skip()
or .Take()
, further filtering must be done in memory.
Order
operations do not guarantee IndexedDB-native ordering.
Final Query Stage – No More IndexedDB Execution Allowed
public interface IMagicQueryFinal<T> : IMagicExecute<T> where T : class
{
Task<IEnumerable<T>> WhereAsync(Expression<Func<T, bool>> predicate);
}
Once you reach this stage, all further filtering happens in memory.
๐ Magic IndexedDBโs system ensures that your queries are optimized for IndexedDB execution wherever possible.
It prevents you from making mistakes that would force everything into memory unnecessarily.
5. Using `.Cursor()` for Full Cursor-Based Queries
If you explicitly want to use a cursor query, use .Cursor()
instead of .Where()
.
✅ Cursor Query Example
await personQuery.Cursor(x => x.Name == "Zack").ToListAsync();
Forces all operations into a cursor query.
Allows deeper filtering than Indexed Queries.
Can still use .OrderBy()
, .Take()
, .Skip()
, etc.
Cursor Query Interface
public interface IMagicCursor<T> : IMagicExecute<T> where T : class
{
IMagicCursor<T> Cursor(Expression<Func<T, bool>> predicate);
IMagicCursorStage<T> Take(int amount);
IMagicCursorSkip<T> Skip(int amount);
}
Cursor queries unlock more capabilities but sacrifice performance.
๐จ Only use .Cursor()
when you know an indexed query wonโt work!
Otherwise, stick with .Where()
for better performance.
6. Supported LINQ Operations
Magic IndexedDB supports a rich LINQ feature set, including:
✅ Comparison Operators
await personQuery.Where(x => x.Age > 30).ToListAsync();
await personQuery.Where(x => x.Name == "Alice").ToListAsync();
✅ String Operations
await personQuery.Where(x => x.Name.StartsWith("C", StringComparison.OrdinalIgnoreCase)).ToListAsync();
await personQuery.Where(x => x.Name.Contains("bo", StringComparison.OrdinalIgnoreCase)).ToListAsync();
await personQuery.Where(x => x.Name.Equals("John", StringComparison.OrdinalIgnoreCase)).ToListAsync();
✅ Deeply Nested `||` Conditions
await personQuery.Where(p =>
(
(p.TestInt == 9 || p.TestInt == 3 || p.TestInt == 7) &&
(
(p.Name == "Luna" || p.Name == "Jerry" || p.Name == "Jamie") ||
(p._Age >= 35 && p._Age <= 40) ||
(p.Name == "Zane" && p._Age > 45)
) &&
(p._Age < 30 || p._Age > 50 || p._Age == 35)
)).ToListAsync();
IndexedDB canโt handle this.
Magic IndexedDB eats it for breakfast. ๐
The universal layer flattens, optimizes, and rewires your predicates without reflection or runtime overhead.
Yes, itโs real. No, itโs not lazy. This is hyper optimized.
You write clean logic โ Magic IndexedDB handles the madness.
Shouldn't this take 720+ operations when flattened?
❓ Mini FAQ — Wait, What?!
๐งฎ Shouldnโt this take like 720+ operations when flattened?
Nope. Magicโs partitioning engine actually collapses this example into just 1 or 2 operations.
๐ But you canโt use Indexed queries with logic like this, right?
Why do you keep thinking there's limitations? This is Magic!
If your schema supports the fields, Magic still uses Indexed queries.
I'm a "make my cake and eat it too" kind of guy โ and Magic IndexedDB is the entire damn bakery.
Executing Queries Directly Without `.Where()` or `.Cursor()`
Instead of using .Where()
or .Cursor()
, you can execute queries directly on the query itself, just like LINQ to SQL.
🔹 Retrieving All Records
await _MagicDb.Query<Person>().ToListAsync();
Fetches the entire table, equivalent to SELECT * FROM Person;
.
🔹 Ordering & Pagination
await _MagicDb.Query<Person>().OrderBy(x => x.Age).Take(5).ToListAsync();
await _MagicDb.Query<Person>().OrderByDescending(x => x.Id).Skip(10).Take(5).ToListAsync();
Supports ordering, skipping, and taking records just like SQL.
Reminder: IndexedDB requires Take()
before Skip()
.
🔹 Fetching a Single Record
await _MagicDb.Query<Person>().FirstOrDefaultAsync();
await _MagicDb.Query<Person>().LastOrDefaultAsync();
Retrieves the first or last record efficiently.
This allows for simple, SQL-like querying while leveraging IndexedDB optimizations behind the scenes! ๐
LINQ to IndexedDB – Full Querying Guide & Operations
1. Understanding Query Operations in Magic IndexedDB
Magic IndexedDB provides a true LINQ to IndexedDB experience, translating LINQ expressions into optimized IndexedDB queries.
There are two primary querying methods:
Query Method | Purpose | Best For |
---|---|---|
.Where(x => Predicate) |
Optimized IndexedDB Queries | Fast queries using indexed fields |
.Cursor(x => Predicate) |
Full Cursor Querying | Advanced filtering when indexing isnโt possible |
TL;DR: Always prefer .Where()
for performance. Use .Cursor()
only when needed.
2. Query Execution – IndexedDB vs. Cursor Queries
Before diving into syntax, it's important to understand how queries execute.
IndexedDB only supports certain query patterns efficiently.
Magic IndexedDB automatically categorizes your query into:
โ Indexed Query
โก Combined Indexed Query
๐ Cursor Query (Meta-data driven)
Each ||
condition is broken down into independent queries.
If an &&
operation cannot be fully indexed, that entire &&
block becomes a Cursor Query.
Using StringComparison.OrdinalIgnoreCase
forces a cursor.
Read the LINQ to IndexedDB Fundamentals to understand how your queries are optimized!
3. Supported LINQ Operations
Magic IndexedDB supports a rich set of LINQ expressions for querying your data.
✅ Comparison Operators
await personQuery.Where(x => x.Age > 30).ToListAsync();
await personQuery.Where(x => x.Name == "Alice").ToListAsync();
✅ String Operations
await personQuery.Where(x => x.Name.StartsWith("C", StringComparison.OrdinalIgnoreCase)).ToListAsync();
await personQuery.Where(x => x.Name.Contains("bo", StringComparison.OrdinalIgnoreCase)).ToListAsync();
await personQuery.Where(x => x.Name.Equals("John", StringComparison.OrdinalIgnoreCase)).ToListAsync();
โ Using StringComparison.OrdinalIgnoreCase
forces a cursor query.
Use indexed fields whenever possible!
✅ Deeply Nested `||` Conditions
await personQuery.Where(p =>
(
(p.TestInt == 9 || p.TestInt == 3 || p.TestInt == 7)
&&
(
(p.Name == "Luna" || p.Name == "Jerry" || p.Name == "Jamie")
|| (p._Age >= 35 && p._Age <= 40)
|| (p.Name == "Zane" && p._Age > 45)
)
&&
(
p._Age < 30 || p._Age > 50 || p._Age == 35
)
)).ToListAsync();
IndexedDB does NOT support deeply nested ||
operations by default.
Magic IndexedDB flattens and optimizes them for you.
4. Query Operations – Order of Execution
The following table defines the operations available and how they interact.
🔹 Indexed Query (.Where()) Execution Order
Operation | Allowed Before | Allowed After | Notes |
---|---|---|---|
.Where() |
Start | .Where() , .ToListAsync() |
Supports indexing optimizations. |
.OrderBy() && order by descending |
.Where() |
.Take() , .Skip() , .ToListAsync() |
Ordering is NOT guaranteed in IndexedDB. Must reapply after query. |
.Take() |
.Where() |
.Skip() , .ToListAsync() |
Must be before .Skip() in IndexedDB. |
.Skip() |
.Take() |
.ToListAsync() |
Skipping before taking ignores take! |
.FirstOrDefaultAsync() |
.Where() |
End | Returns first matching record. |
.LastOrDefaultAsync() |
.Where() |
End | Returns last matching record. |
.StableOrdering() |
.Cursor() |
End | Strict Ordering (click to learn more) |
🔹 Cursor Query (.Cursor()) Execution Order
Operation | Allowed Before | Allowed After | Notes |
---|---|---|---|
.Cursor() |
Start | .OrderBy() , .Take() , .Skip() , .ToListAsync() |
Allows full flexibility but is slower. |
.OrderBy() |
.Cursor() |
.Take() , .Skip() , .ToListAsync() |
Order will not be enforced at IndexedDB level. |
.TakeLast() |
.OrderBy() |
.ToListAsync() |
Forces in-memory filtering. |
.Skip() |
.Take() |
.ToListAsync() |
Always apply .Take() before .Skip() . |
.FirstOrDefaultAsync() |
.Cursor() |
End | Returns first matching record. |
.LastOrDefaultAsync() |
.Cursor() |
End | Returns last matching record. |
๐จ Once you use .Skip()
, .Take()
, .OrderBy()
, filtering becomes memory-based!
IndexedDB does not allow additional .Where()
operations after these.
📌 Supported Query Operators
Operator (C# / LINQ Style) | IndexedDB Optimized? |
---|---|
== |
โ Yes |
!= |
โ Yes |
> |
โ Yes |
>= |
โ Yes |
< |
โ Yes |
<= |
โ Yes |
.StartsWith() |
โ Yes* (case-insensitive uses cursor) |
!x.StartsWith() |
๐ซ Cursor Required |
.EndsWith() |
๐ซ Cursor Required |
!x.EndsWith() |
๐ซ Cursor Required |
.Contains() |
โ Yes* (not for strings) |
!x.Contains() |
โ Yes* (not for strings) |
.In([a, b, c]) |
โ Yes |
.Length == X |
๐ซ Cursor Required |
.Length > X / >= X / < X / <= X |
๐ซ Cursor Required |
x == null |
๐ซ Cursor Required |
x != null |
๐ซ Cursor Required |
*Contains for non-strings โ when working with arrays, the system tries to flatten the predicate to the universal language on your behalf.
🕒 Date & Time Query Support (C# Style)
C# Expression | IndexedDB Optimized? |
---|---|
x == DateTime(2020, 5, 1) | โ Yes (translated to range) |
x != DateTime(2020, 5, 1) | ๐ซ Cursor Required |
x > DateTime(2023, 1, 1) | โ Yes |
x >= DateTime(2023, 1, 1) | โ Yes |
x < DateTime(2023, 1, 1) | โ Yes |
x <= DateTime(2023, 1, 1) | โ Yes |
x.Date == new DateTime(2023, 10, 15) | โ Yes (translated to range) |
x.Year == 2023 | โ Yes |
x.Year != 2023 | ๐ซ Cursor Required |
x.Year > 2022 | โ Yes |
x.Year >= 2023 | โ Yes |
x.Year < 2024 | โ Yes |
x.Year <= 2023 | โ Yes |
x.Month == 7 | ๐ซ Cursor Required |
x.Month != 12 | ๐ซ Cursor Required |
x.Month > 6 | ๐ซ Cursor Required |
x.Month >= 1 | ๐ซ Cursor Required |
x.Month < 12 | ๐ซ Cursor Required |
x.Month <= 7 | ๐ซ Cursor Required |
x.Day == 4 | ๐ซ Cursor Required |
x.Day != 5 | ๐ซ Cursor Required |
x.Day > 2 | ๐ซ Cursor Required |
x.Day >= 10 | ๐ซ Cursor Required |
x.Day < 20 | ๐ซ Cursor Required |
x.Day <= 15 | ๐ซ Cursor Required |
x.DayOfWeek == DayOfWeek.Monday | ๐ซ Cursor Required |
x.DayOfWeek != DayOfWeek.Friday | ๐ซ Cursor Required |
x.DayOfWeek > DayOfWeek.Sunday | ๐ซ Cursor Required |
x.DayOfWeek >= DayOfWeek.Tuesday | ๐ซ Cursor Required |
x.DayOfWeek < DayOfWeek.Saturday | ๐ซ Cursor Required |
x.DayOfWeek <= DayOfWeek.Monday | ๐ซ Cursor Required |
x.DayOfYear == 128 | ๐ซ Cursor Required |
x.DayOfYear != 129 | ๐ซ Cursor Required |
x.DayOfYear > 50 | ๐ซ Cursor Required |
x.DayOfYear >= 100 | ๐ซ Cursor Required |
x.DayOfYear < 200 | ๐ซ Cursor Required |
x.DayOfYear <= 365 | ๐ซ Cursor Required |
✅ BONUS: Nullable Support
x.DateOfBirth.Value
(nullable structs)
Automatic .Value
stripping where safe
Error-handling for invalid usage like x.DateOfBirth.Value
(without member access)
💡 Pro Tip
Operations like .Date
, .Year
, .Month
, .Day
, .DayOfWeek
, and .DayOfYear
are all internally optimized and recognized as special operations โ no reflection, no guesswork, no slowdowns.
This is next-gen IndexedDB querying.
🔎 Type-Based Queries
Operator | IndexedDB Optimized? |
---|---|
x is type of int/number | ๐ซ Cursor Required |
x is type of string | ๐ซ Cursor Required |
typeof x === "number" | ๐ซ Cursor Required |
typeof x === "string" | ๐ซ Cursor Required |
x is DateTime / Date | ๐ซ Cursor Required |
x is Array | ๐ซ Cursor Required |
x is Object | ๐ซ Cursor Required |
x is Blob | ๐ซ Cursor Required |
x is ArrayBuffer | ๐ซ Cursor Required |
x is File | ๐ซ Cursor Required |
*Type of both equals and not equals supported.
The system automatically detects what can or cannot be converted to a JS type since IndexedDB is JavaScript-based. So type checking is not as strict as C#.
5. Cursor Queries – Unlocking Full IndexedDB Power
A .Cursor()
query forces everything into a cursor-based scan, removing restrictions but also removing optimizations.
✅ Cursor Query Example
await personQuery.Cursor(x => x.Name == "Zack").ToListAsync();
Forces all operations into a cursor query.
Unlocks .TakeLast()
, .Skip()
, and .OrderBy()
.
Can be combined with .Where()
, but will convert entire query into a cursor.
6. Query Interface Pathways
The following flowchart defines the order in which query operations are enforced.
🔹 Query Flowchart
Start โ Where() โ OrderBy() โ Take() โ Skip() โ Execution (.ToListAsync())
โ Cursor() โ TakeLast() โ Execution
๐ฅ Magic IndexedDB enforces these rules at the interface level!
You canโt accidentally write non-performant IndexedDB queries.
7. Full Query Examples
// Basic WHERE statement with optimized indexing
await personQuery.Where(x => x.Age > 30).ToListAsync();
// WHERE with OR conditions (flattened optimization)
await personQuery.Where(x => (x.Age > 40 && x.TestInt == 9) || x.Name.Contains("bo")).ToListAsync();
// ORDER BY, TAKE, and SKIP (Indexed)
await personQuery.Where(x => x.Age > 30)
.Take(3)
.Skip(2)
.ToListAsync();
// Cursor-based querying (Allows `.TakeLast()`)
await personQuery.Cursor(x => x.Age > 30)
.OrderBy(x => x.Age)
.TakeLast(2)
.ToListAsync();
// FirstOrDefault and LastOrDefault
await personQuery.OrderBy(x => x.Age).FirstOrDefaultAsync();
await personQuery.OrderBy(x => x.Age).LastOrDefaultAsync();
Quick "good to know"
Use Contains for both strings and arrays:
int[] myArray = { 38, 39 };
await personQuery.Where(x => myArray.Contains(x._Age));
This works for arrays in both directions.
.Cursor(x => Your_Predicate) allows different paths than .Where and supports current and future planned operations like .Min, .Max, and .Select.
Biggest TLDR of why .Cursor is different from .Where is that when using .Cursor, there is no way an indexed query will fire. The .Where doesnโt guarantee that an indexed query fires, but it means itโs not impossible.
Utilizing capabilities like x.Item == null or x.Item != null is fully supported.
But itโs important to remember this translation checks if itโs directly set to null, an empty string (if it's a string), or an undefined column.
Avoid querying with x.Item.Value on nullable variables when possible.
It can throw the serializer off. For supported appended operations like .Length, .Year, .GetDay, .GetDayOfWeek, .GetDayOfYear, these and a few others are the only times you should use the .Value suffix. This is the only way in C# to allow access to such properties on nullable types (e.g., x.Item.Value.Length).
What You Need To Know
Before you go off and start using the library. This documentation was for sure the bulkiest, but everything else is much smaller! You've done it, congrats! But there's still really critical information you must be aware of. Before you venture forth, I suggest reading the following in order:
Read the Utility Guide To learn about the additional Syntax and capabilities.
Read about .Cursor() vs .Where() To learn about why .Cursor() vs .Where() exist separately.
How Ordering Works To learn about how and why the ordering is different with LINQ to IndexedDB.
Installation Instructions To learn about how to setup your project.
Migrations Guide To learn about how to setup the migrations for future updates.
Read the LINQ to IndexedDB Fundamentals to master IndexedDB optimizations! ๐
It's not as much as you'd think, I promise! It's worth knowing and understanding.