LINQ To IndexedDB - Magic IndexedDB
Author: Lance Wright II
Published: March 25, 2025
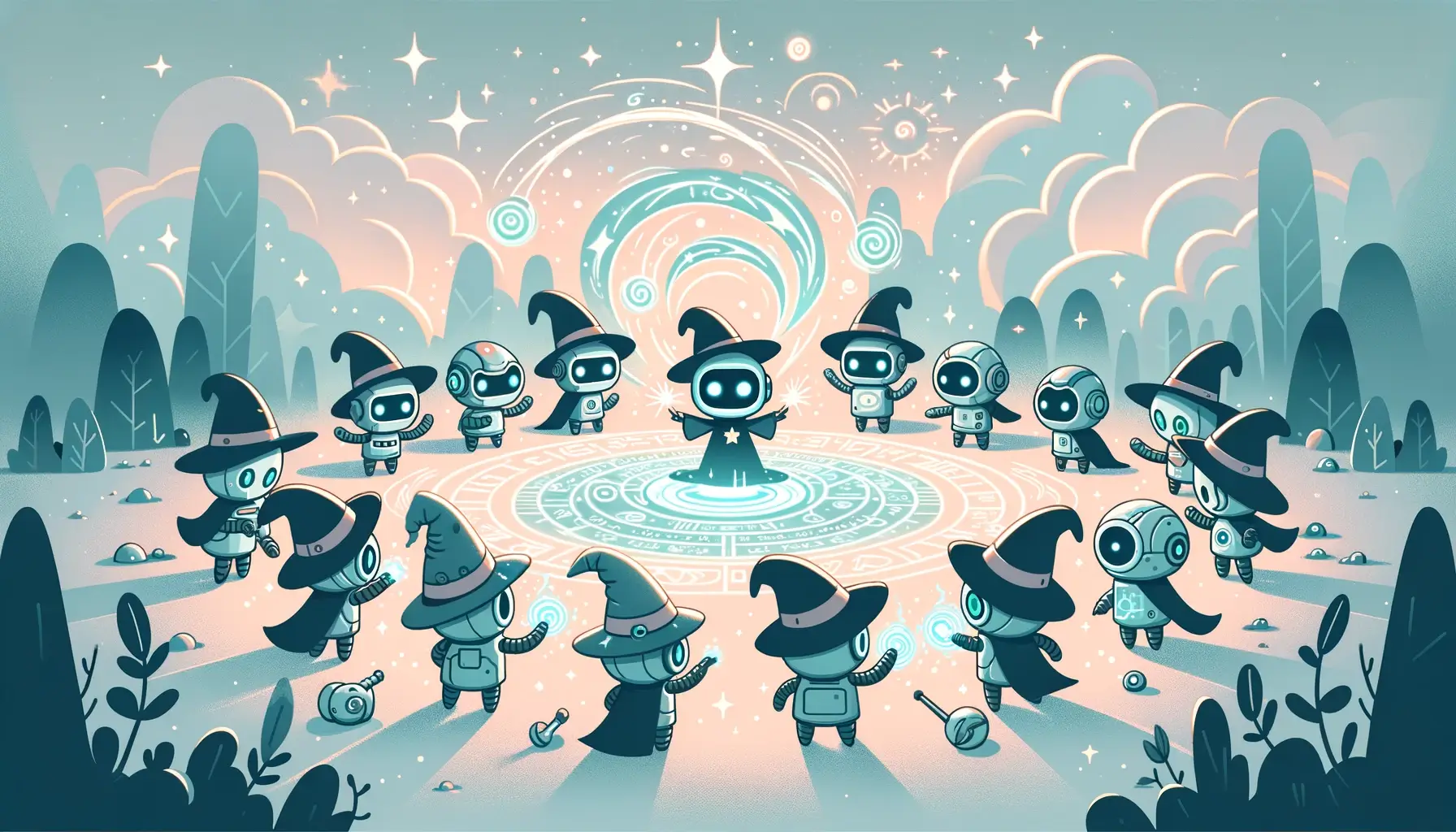
Cookies? Service Workers? Cute. Here's a Nuclear Engine.
Call me a priest — I’ve exorcised IndexedDB.
Call me a mad scientist — I wired a full query engine into a storage system the world gave up on.
And it works — God help us, it works.
IndexedDB: Powerful. Untouchable. No Longer.
You know the truth.
IndexedDB is the most powerful browser-native storage ever made. It’s also been a total disaster to use.
- The API is a fever dream.
- The docs are a cruel joke.
- Migrations are near impossible to juggle.
- And every wrapper out there? Just sweetened trash.
So you did what everyone did:
- Used cookies for state.
- LocalStorage for user prefs.
- Service worker caches as a poor man’s data layer.
- Built fragile custom sync logic to fake offline capability.
All because IndexedDB was too raw, too low-level, and way too painful.
Not anymore.
True Translated Intent
This isn’t lipstick on a storage pig
This is a complete query engine, running inside the browser.
And I mean engine — with execution trees, deferred logic, metadata introspection, and tons of optimizations.
Csharp Blazor example, but hint hint. This is useable and ready for every language.
IMagicQuery<Person> personQuery = await _MagicDb.Query<Person>();
List<Person> persons = new List<Person>
{
new Person { Name = "Alice", Age = 25 },
new Person { Name = "Bob", Age = 28 }
};
await personQuery.AddRangeAsync(persons);
await personQuery
.Where(x => x.Age >= 26 && x.Name.StartsWith("aL", StringComparison.OrdinalIgnoreCase) || x.Name.Length > 3)
.OrderByDescending(x => x.LastLogin.Value.Year)
.Take(25)
.Skip(10)
.ToListAsync();
Yes, that runs. Yes, inside IndexedDB.
No tricks. No compilation. Just pure LINQ, end to end.
You can:
- Use || and && like a full-grown adult
- Dive into nested && ||
- Run .Length on strings and arrays
- Combine filters with OrderBy, Skip, Take, .Count(), .FirstOrDefault(), and more!
- Stream results without loading a single item into memory unless you ask for it
This is not a filter layered on top of a fetch.
This _is_ a query engine.
And that engine is partition-aware, metadata-driven, and damn smart.
It knows what can be indexed.
What needs to fall back.
How to minimize memory pressure.
How to optimize execution paths dynamically — while you sleep.
This is the kind of technology that makes your grandmother think you're playing with black magic. But... Maybe she's not wrong?
A Real Engine, Not a Wrapper
I didn’t just "wrap" IndexedDB.
I built a universal language, predicate aware, intent based query engine. I know, I know. Tons of fancy words here.
Every query becomes a serialized predicate tree, handed off to a partition-aware execution layer that knows:
- What can be satisfied by pure index calls
- What needs the cursor engine metadata driven fallback
- What must be merged, scoped, and executed with precision
- What each executed operation must do while running in parallel to result as if it was a single query
You don’t write “IndexedDB logic.”
You write what you want, and Magic IndexedDB handles the rest.
And no, we never bulk-fetch.
We never blindly iterate.
Nothing enters memory unless it's truly needed.
This thing is smarter than your average ORM.
And it’s running inside your browser.
Built Once. Runs Everywhere.
You saw the C# code. But this isn't just for Blazor.
This is due to the powers of the Universal Predicate Protocol — a cross-language serialization layer that turns LINQ into a clean, nestable, index-aware instruction tree. Portable. Extensible. Weaponized.
That means Magic IndexedDB supports:
- JavaScript
- Rust
- Python
- GO
- Any platform with IndexedDB
This is a protocol, not a helper. One engine between frameworks. Universal, shareable, unique, yet can be powered and empower by every community.
This is how IndexedDB should have worked from the start.
And Did I Mention Migrations?
Yeah, it does that too.
But not like you’re used to.
Forget the brittle version bumps and schema hell. This thing can:
- Detect schema changes
- Drop in tables dynamically between databases
- Remove dead schema cleanly and safely
- Automatic scaffolding of changes
- So so so much more.
Migration shouldn’t be a monster. It should just work.
So I made it work.
Magic? Maybe.
I don’t blame you if it feels like dark sorcery. Especially if you're already familiar with IndexedDB. I mean how can you have:
- True LINQ-to-IndexedDB translation.
- Be fully async.
- Be universally portable.
- Parallel intent based query-optimized operations.
- Achieve memory-efficiency to never load anything in memory across parallel executions.
- Cross language deferred by default.
- No third-party sync services.
- No smoke. No mirrors.
The Secret - Mic Drop
IndexedDB was never the problem.
The tools and philosophy were.
Why MIT License Free? Why So Good?
There are so many companies that generically claim they're innovators, specialized, blah blah blah. But at Sayou, we have the ambitions of a madman—a vision of a world with no boundaries between technologies. A future where data flows as easily as rivers carve their path to the ocean.
Magic IndexedDB was just one of many so-called “impossible” problems we’ve already solved on our path toward something greater. We challenge industry standards. We break rules that deserve breaking. And the projects we’re about to release? They'll shake the foundation of what's considered possible.
If you need a developer, we’re not the company for you. If you need tactical consultation, architectural protocol design, or strategic innovation at the bleeding edge—then reach out. Because our mission hasn’t changed since day one:
We are here to pioneer.
And it all starts with listening.
So—what say you?
Getting Started - Links/Guide
Ready to get started with the web storage of the future? Magic IndexedDB has guides and documentation to get you started!