Magic IndexedDB – Universal Translation Layer
Magic IndexedDB – Universal Translation Layer
Author: Lance Wright II
Published: March 26, 2025
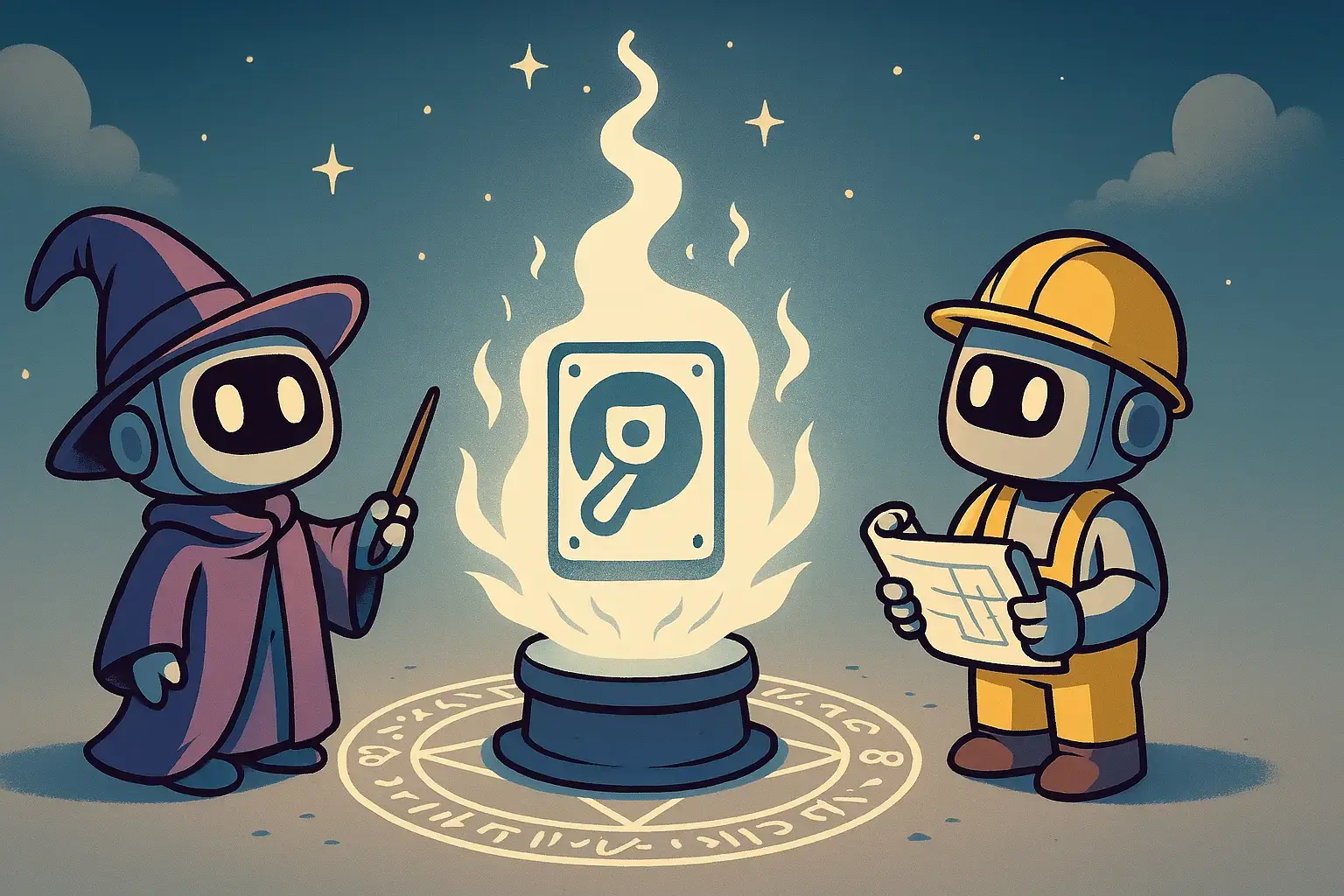
Magic IndexedDB – Universal Translation Layer
Bringing true LINQ to IndexedDB to any framework, language, or platform!
1. What is the Universal Translation Layer?
Magic IndexedDB isn’t just a Blazor library—it’s a fully universal LINQ to IndexedDB translation system that any language or framework can hook into.
Instead of directly wrapping IndexedDB APIs, Magic IndexedDB acts as a middleware layer, translating LINQ-based queries into optimized IndexedDB requests using Dexie.js as the underlying IndexedDB wrapper.
Why Use Dexie.js?
✅ Mature & well-supported library
✅ Optimized IndexedDB access
✅ Battle-tested by thousands of developers
✅ Prevents reinventing the wheel—Magic IndexedDB focuses on the translation, not the direct API
🚀 The Goal: Build a universal IndexedDB querying system any language can implement.
2. How Queries Are Translated
Magic IndexedDB converts LINQ-style operations into a structured, universal query format, optimizing IndexedDB interactions wherever possible. However, due to IndexedDB's inherent limitations, certain operations must be handled using cursors instead of direct indexed queries.
📌 Supported Query Operators
🧮 Basic Comparison
Operator | JavaScript Key | Description | IndexedDB Optimized? |
---|---|---|---|
== | Equal | Exact match | ✅ Yes |
!= | NotEqual | Not equal | ✅ Yes |
> | GreaterThan | Greater than | ✅ Yes |
>= | GreaterThanOrEqual | Greater than or equal | ✅ Yes |
< | LessThan | Less than | ✅ Yes |
<= | LessThanOrEqual | Less than or equal | ✅ Yes |
🔤 String Matching
Operator | JavaScript Key | Description | IndexedDB Optimized? |
---|---|---|---|
.startsWith() | StartsWith | String starts with | ✅ Yes* (case-insensitive = 🚫) |
!x.startsWith() | NotStartsWith | Does not start with | 🚫 Cursor Required |
.endsWith() | EndsWith | String ends with | 🚫 Cursor Required |
!x.endsWith() | NotEndsWith | Does not end with | 🚫 Cursor Required |
.contains() | Contains | String/array contains | 🚫 Cursor Required |
!x.contains() | NotContains | Does not contain | 🚫 Cursor Required |
🚨 Important Notes:
✅ Indexed queries are optimized, but operations like .Contains()
always require a cursor scan.
✅ StartsWith()
is indexable if the comparison is case-sensitive (caseSensitive: true
in the universal layer).
⚠️ If StringComparison.OrdinalIgnoreCase
or caseSensitive: false
is used, cursor fallback will occur.
🧪 This may not be everything—validate against QUERY_OPERATIONS
in queryConstants.js
.
📚 Array & Length Operations
Operator | JavaScript Key | Description | IndexedDB Optimized? |
---|---|---|---|
.In([a, b, c]) | In | Matches any value in array | ✅ Yes |
.length == X | LengthEqual | Length equals X | 🚫 Cursor Required |
.length != X | NotLengthEqual | Length not equal to X | 🚫 Cursor Required |
.length > X | LengthGreaterThan | Length greater than X | 🚫 Cursor Required |
.length >= X | LengthGreaterThanOrEqual | Greater or equal to X | 🚫 Cursor Required |
.length < X | LengthLessThan | Length less than X | 🚫 Cursor Required |
.length <= X | LengthLessThanOrEqual | Less than or equal to X | 🚫 Cursor Required |
📅 Date Operations
Operator | JavaScript Key | IndexedDB Optimized? |
---|---|---|
x.Month == 7 | MonthEqual | 🚫 Cursor Required |
x.Month != 7 | NotMonthEqual | 🚫 Cursor Required |
x.Month > 7 | MonthGreaterThan | 🚫 Cursor Required |
x.Month >= 7 | MonthGreaterThanOrEqual | 🚫 Cursor Required |
x.Month < 7 | MonthLessThan | 🚫 Cursor Required |
x.Month <= 7 | MonthLessThanOrEqual | 🚫 Cursor Required |
x.Day == 15 | DayEqual | 🚫 Cursor Required |
x.Day != 15 | NotDayEqual | 🚫 Cursor Required |
x.Day > 15 | DayGreaterThan | 🚫 Cursor Required |
x.Day >= 15 | DayGreaterThanOrEqual | 🚫 Cursor Required |
x.Day < 15 | DayLessThan | 🚫 Cursor Required |
x.Day <= 15 | DayLessThanOrEqual | 🚫 Cursor Required |
x.DayOfWeek == X | DayOfWeekEqual | 🚫 Cursor Required |
x.DayOfWeek != X | NotDayOfWeekEqual | 🚫 Cursor Required |
x.DayOfWeek > X | DayOfWeekGreaterThan | 🚫 Cursor Required |
x.DayOfWeek >= X | DayOfWeekGreaterThanOrEqual | 🚫 Cursor Required |
x.DayOfWeek < X | DayOfWeekLessThan | 🚫 Cursor Required |
x.DayOfWeek <= X | DayOfWeekLessThanOrEqual | 🚫 Cursor Required |
x.DayOfYear == 128 | DayOfYearEqual | 🚫 Cursor Required |
x.DayOfYear != 128 | NotDayOfYearEqual | 🚫 Cursor Required |
x.DayOfYear > 128 | DayOfYearGreaterThan | 🚫 Cursor Required |
x.DayOfYear >= 128 | DayOfYearGreaterThanOrEqual | 🚫 Cursor Required |
x.DayOfYear < 128 | DayOfYearLessThan | 🚫 Cursor Required |
x.DayOfYear <= 128 | DayOfYearLessThanOrEqual | 🚫 Cursor Required |
x.Year == 2024 | YearEqual | ✅ Yes |
x.Year != 2024 | NotYearEqual | 🚫 Cursor Required |
x.Year > 2024 | YearGreaterThan | ✅ Yes |
x.Year >= 2024 | YearGreaterThanOrEqual | ✅ Yes |
x.Year < 2024 | YearLessThan | ✅ Yes |
x.Year <= 2024 | YearLessThanOrEqual | ✅ Yes |
🧪 Type Checks
Operator | JavaScript Key | Description | IndexedDB Optimized? |
---|---|---|---|
typeof x === "number" | TypeOfNumber | Value is a number | 🚫 Cursor Required |
typeof x === "string" | TypeOfString | Value is a string | 🚫 Cursor Required |
x instanceof Date | TypeOfDate | Value is a valid Date | 🚫 Cursor Required |
Array.isArray(x) | TypeOfArray | Value is an array | 🚫 Cursor Required |
typeof x === "object" | TypeOfObject | Value is a plain object | 🚫 Cursor Required |
x instanceof Blob | TypeOfBlob | Value is a Blob | 🚫 Cursor Required |
x instanceof ArrayBuffer | TypeOfArrayBuffer | Value is an ArrayBuffer or typed array | 🚫 Cursor Required |
x instanceof File | TypeOfFile | Value is a File | 🚫 Cursor Required |
!(typeof x === "number") | NotTypeOfNumber | Value is not a number | 🚫 Cursor Required |
!(typeof x === "string") | NotTypeOfString | Value is not a string | 🚫 Cursor Required |
!(x instanceof Date) | NotTypeOfDate | Value is not a valid Date | 🚫 Cursor Required |
!Array.isArray(x) | NotTypeOfArray | Value is not an array | 🚫 Cursor Required |
!(typeof x === "object") | NotTypeOfObject | Value is not a plain object | 🚫 Cursor Required |
!(x instanceof Blob) | NotTypeOfBlob | Value is not a Blob | 🚫 Cursor Required |
!(x instanceof ArrayBuffer) | NotTypeOfArrayBuffer | Value is not an ArrayBuffer or typed array | 🚫 Cursor Required |
!(x instanceof File) | NotTypeOfFile | Value is not a File | 🚫 Cursor Required |
🚫 Null Checks
Operator | JavaScript Key | Description | IndexedDB Optimized? |
---|---|---|---|
x == null | IsNull | Value is null or undefined | 🚫 Cursor Required |
x != null | IsNotNull | Value is not null or undefined | 🚫 Cursor Required |
📌 Query Additions (Sorting & Pagination)
LINQ Operation | JavaScript Key | Description |
---|---|---|
.StableOrdering() | stableOrdering | Predictable Ordering |
.OrderBy() | orderBy | Sort ascending |
.OrderByDescending() | orderByDescending | Sort descending |
.FirstOrDefaultAsync() | first | Get first item |
.LastOrDefaultAsync() | last | Get last item |
.Skip(x) | skip | Skip x results |
.Take(x) | take | Take x results |
.TakeLast(x) | takeLast | Take last x results |
⚠ IndexedDB has a reversed order for .Take()
and .Skip()
!
Always write .Take()
before .Skip()
for correct execution.
3. How Queries Are Structured
To integrate any language or framework, you need to convert expressions into the universal query format.
Example of a Universal Query Structure
{
"nodeType": "logical",
"operator": "And",
"children": [
{
"nodeType": "condition",
"condition": {
"property": "age",
"operation": "GreaterThan",
"value": 30,
"isString": false,
"caseSensitive": false
}
},
{
"nodeType": "logical",
"operator": "Or",
"children": [
{
"nodeType": "condition",
"condition": {
"property": "city",
"operation": "Equal",
"value": "New York"
}
},
{
"nodeType": "condition",
"condition": {
"property": "city",
"operation": "Equal",
"value": "San Francisco"
}
}
]
}
]
}
This query is equivalent to:
await personQuery
.Where(x => x.Age > 30 && (x.City == "New York" || x.City == "San Francisco"))
.ToListAsync();
🔍 Overview: Universal Predicate Language (UPL)
Purpose:
This format allows any programming language to serialize complex, nested logical filters (AND/OR) into a universal, portable structure that can be interpreted by any system (like your Magic IndexedDB engine, SQL, NoSQL, etc.).
🌲 Visual Map Example (for the JSON you provided)
AND
├── CONDITION: age > 30
└── OR
├── CONDITION: city == "New York"
└── CONDITION: city == "San Francisco"
This shows:
📌 A top-level AND
📌 The left child is a condition (age > 30
)
📌 The right child is a nested OR with two conditions
🧱 JSON Structure Explained
type PredicateNode =
| LogicalNode
| ConditionNode;
interface LogicalNode {
nodeType: "logical";
operator: "And" | "Or";
children: PredicateNode[];
}
interface ConditionNode {
nodeType: "condition",
condition: {
property: string,
operation: "Equal" | "NotEqual" | "GreaterThan" | "LessThan" | ...,
value: any,
isString?: boolean,
caseSensitive?: boolean
}
}
This schema lets you nest as deeply as needed, mixing ANDs and ORs, enabling arbitrarily complex logic trees.
🛠️ Use Case: Translating Native Predicates
Here’s how to implement this in your language:
C# (LINQ)
x => x.Age > 30 && (x.City == "New York" || x.City == "San Francisco")
Becomes:
{
"nodeType": "logical",
"operator": "And",
"children": [
{
"nodeType": "condition",
"condition": {
"property": "Age",
"operation": "GreaterThan",
"value": 30
}
},
{
"nodeType": "logical",
"operator": "Or",
"children": [
{
"nodeType": "condition",
"condition": {
"property": "City",
"operation": "Equal",
"value": "New York"
}
},
{
"nodeType": "condition",
"condition": {
"property": "City",
"operation": "Equal",
"value": "San Francisco"
}
}
]
}
]
}
📘 Reference: Supported Operations
Operation | Meaning |
---|---|
Equal | x == value |
NotEqual | x != value |
GreaterThan | x > value |
LessThan | x < value |
Contains | x contains value (string) |
In | x in [value1, value2] |
StartsWith | x starts with value |
EndsWith | x ends with value |
There's a lot more operations than this built in. Documentation of the universal translation layer is still being updated.
🧠 Developer Notes
You can nest any combination of AND and ORs.
Each logical
node can have any number of children (not just 2).
Supports easy translation to/from:
— LINQ expressions
— SQL WHERE clauses
— JavaScript Array.filter
chains
— MongoDB query documents
You can build a universal query editor UI using this structure.
The universal translation layer handles complex nested (&&) and (||) operations for you with flattening, optimizations, and more. This is how Magic IndexedDB can perform complex nested operations while IndexedDB by default cannot.
4. IndexedDB Store Structure (DB Schema & Configuration)
IMPORTANT NOTE:
This section for the structure of the DB Schema is highly likely to change until the migration code is completely released. The future migration implementation will require adjustments here.
Before executing queries, Magic IndexedDB must understand how your database is structured. The system defines database stores dynamically based on schemas, ensuring proper indexing, compound keys, and validation for optimized queries.
📌 Example of a DB Store Schema
{
"name": "MyDatabase",
"version": 1,
"storeSchemas": [
{
"tableName": "Users",
"primaryKeyAuto": false,
"columnNamesInCompoundKey": ["UserId", "TenantId"],
"uniqueIndexes": ["Email"],
"indexes": ["FirstName", "LastName", "CreatedAt"],
"columnNamesInCompoundIndex": [[ "LastName", "FirstName" ]]
},
{
"tableName": "Orders",
"primaryKeyAuto": true,
"columnNamesInCompoundKey": ["OrderId"],
"uniqueIndexes": [],
"indexes": ["UserId", "Status"],
"columnNamesInCompoundIndex": [[ "UserId", "Status" ]]
}
]
}
🔍 Breakdown of Each Property
Property | Type | Description |
---|---|---|
name | string | Name of the database |
version | number | Database version (used for migrations) |
storeSchemas | array | List of table definitions |
storeSchemas[].tableName | string | Name of the table (IndexedDB object store) |
storeSchemas[].primaryKeyAuto | boolean | Whether the primary key auto-increments |
storeSchemas[].columnNamesInCompoundKey | array<string> | Columns forming a compound primary key |
storeSchemas[].uniqueIndexes | array<string> | Columns with unique constraints |
storeSchemas[].indexes | array<string> | Indexable columns for optimized queries |
storeSchemas[].columnNamesInCompoundIndex | array<array<string>> | Compound indexes for faster lookups |
🚀 Why This Matters:
The schema is critical for ensuring that indexed queries work properly.
Unique indexes prevent duplicate values in specific fields (e.g., Email
).
Compound indexes allow multi-column optimizations for advanced filtering.
If your database schema is not properly structured, queries may default to slower cursor-based scans instead of optimized index lookups.
5. Executing Queries
Once validated, queries are executed using Dexie.js and Magic IndexedDB’s optimized universal translation layer.
Yield-Based Query Execution
export async function* magicQueryYield(dbName, storeName, nestedOrFilter, queryAdditions = [], forceCursor = false) {
if (!isValidFilterObject(nestedOrFilter)) {
throw new Error("Invalid filter object provided.");
}
if (!isValidQueryAdditions(queryAdditions)) {
throw new Error("Invalid query additions.");
}
// Execute Dexie.js query
}
🔹 Queries use yield
to return results as they arrive.
🔹 A non-yield version (magicQueryAsync
) is also available.
6. Integrating Your Own Framework
To integrate a new language or framework, follow these steps:
Step 1: Convert Expressions into Universal Query Format
🔄 Parse your language’s LINQ or query expressions.
🧩 Map them to QUERY_OPERATIONS
and QUERY_ADDITIONS
.
Step 2: Send Queries to the Universal Layer
⚙ Use magicQueryAsync()
or magicQueryYield()
to execute queries.
Step 3: Handle Results
⏳ Use async iteration (for await
) for streaming data.
📦 Use bulk fetching (magicQueryAsync()
) for standard queries.
7. Database & Table Handling
This process is still under development.
8. Contributing to the Universal Layer
If you're building a wrapper for a new framework:
🔧 Fork the repository.
📬 Submit a PR with your integration.
🌍 Join the community!
🔥 The dream? A universal LINQ to IndexedDB library for every programming language!
Let's make LINQ to IndexedDB a thing for all languages. Let’s make a better internet together.
9. Summary
✅ Magic IndexedDB is a universal LINQ to IndexedDB system.
✅ Supports multiple languages & frameworks through its universal query format.
✅ Validation & translation ensure queries are safe & optimized.
✅ Dexie.js powers efficient IndexedDB interactions.
✅ Integrate your framework with a simple expression parser!