Database Management and Utility Functions in Magic IndexedDB
Database Management and Utility Functions in Magic IndexedDB
Author: Lance Wright II
Published: March 26, 2025
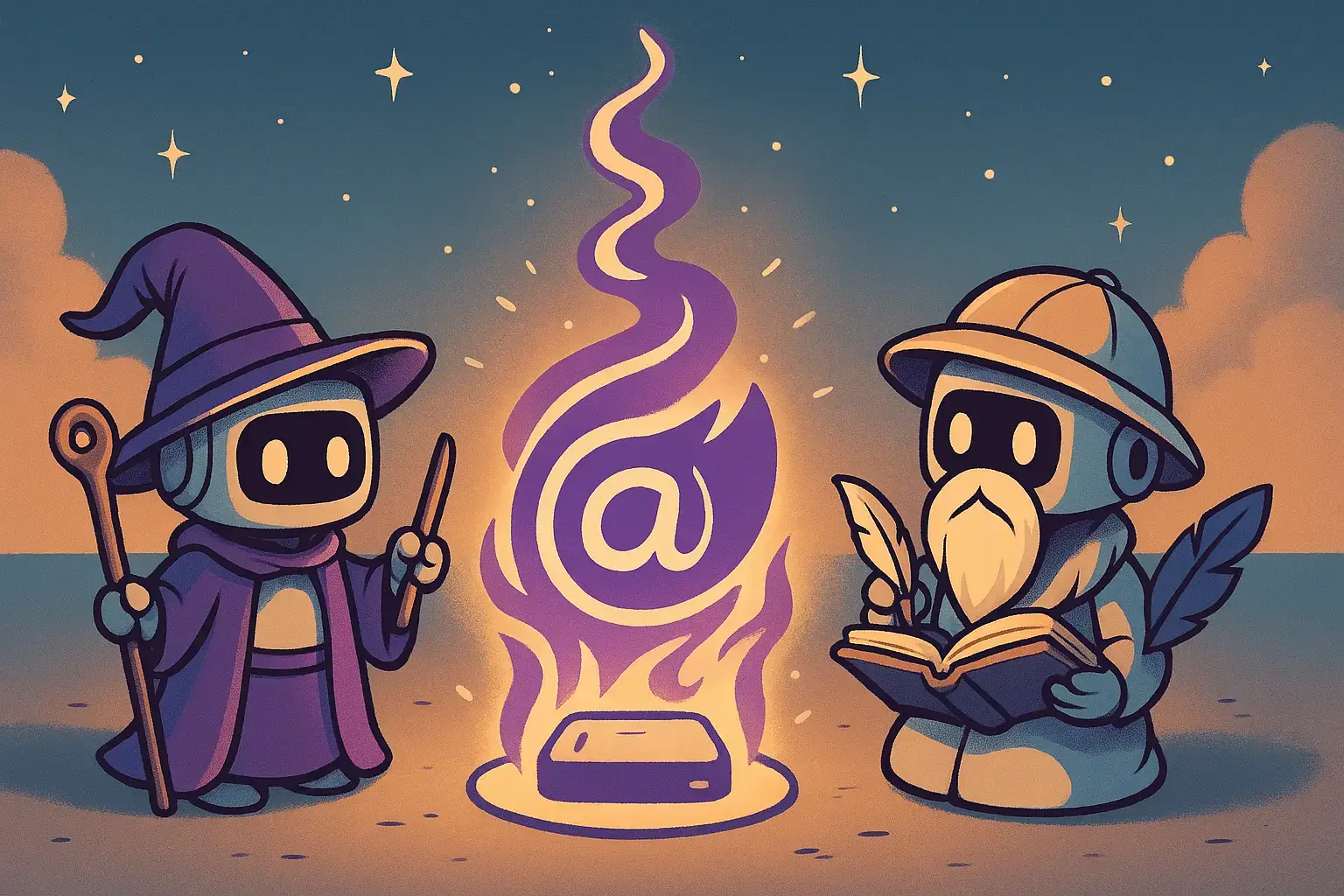
Database Management and Utility Functions in Magic IndexedDB
📌 Understanding Database Deployment in Magic IndexedDB
One of the key design principles behind Magic IndexedDB is its "Table First" approach. Unlike traditional database-first or schema-first approaches, Magic IndexedDB treats tables as the primary focus, while databases act as a means to organize them rather than defining strict boundaries.
🔥 What Does This Mean?
✅ Databases are automatically deployed when a table is queried for the first time.
✅ No explicit deployment syntax is required—simply querying a table will ensure the database exists.
✅ Database versions are a means to an end, allowing table definitions to remain flexible.
🚀 Dynamic Database Handling
When calling:
IMagicQuery<Person> personQuery = await _MagicDb.Query<Person>();
If Person
has never been deployed, Magic IndexedDB automatically deploys it in its assigned database.
If Person
already exists, the database opens automatically for querying.
No additional setup is needed—Magic IndexedDB handles database and table initialization seamlessly.
⚡ Using Magic IndexedDB Without Migrations
Magic IndexedDB provides a powerful auto-migration system via Table Snapshots and Scaffolding CLI.
However, if you choose NOT to use migrations, you must be aware of the following constraints:
1️⃣ Tables Must Be Predefined to Specific Databases
Without migrations, tables must be explicitly assigned to databases at compile time.
✅ Safe Queries With Defined Database Connections
IMagicQuery<Person> personQuery = await _MagicDb.Query<Person>(); // Default database
IMagicQuery<Person> employeeDbQuery = await _MagicDb.Query<Person>(x => x.Databases.Client);
Both queries are safe because Person
is predefined within its respective database.
⚠️ Risky Queries – Unassigned Database Queries Are Not Allowed
🚨 Future Feature - This feature isn't fully implemented yet and has been disabled.
IMagicQuery<Person> animalDbQuery = await _MagicDb.Query<Person>(IndexDbContext.Animal);
🚨 This is NOT allowed without migrations!
Person
is NOT assigned to Animal
, so this query will cause runtime issues.
You must use migrations to support this level of flexibility.
2️⃣ Schema Mismatches Will Trigger Full Database Redeployment
When table structures do not match the expected schema, Magic IndexedDB automatically deletes and redeploys the entire database.
This happens because migrations are not active, meaning manual migration handling is impossible.
🔹 Managing Databases with _MagicDb.Database()
While Magic IndexedDB automatically handles database deployment, you can still manually manage databases when needed.
📌 Closing & Deleting a Single Database
await _MagicDb.Database(IndexDbContext.Animal).Close(); // Closes Animal DB
await _MagicDb.Database(IndexDbContext.Animal).Delete(); // Deletes Animal DB
Closing a database will disconnect all active queries but will reopen it automatically if queried again.
Deleting a database will permanently remove all stored data.
📌 Closing & Deleting Multiple Databases
await _MagicDb.Database(IndexDbContext.Animal, IndexDbContext.Client).Close(); // Closes multiple databases
await _MagicDb.Database(IndexDbContext.Animal, IndexDbContext.Client).Delete(); // Deletes multiple databases
You can target multiple databases in a single operation.
All specified databases will be closed or deleted simultaneously.
📌 Closing & Deleting All Databases
await _MagicDb.Database().CloseAll(); // Closes all databases
await _MagicDb.Database().DeleteAll(); // Deletes all databases
No parameters = affects all deployed databases.
Useful for cleanup operations when resetting IndexedDB storage.
⚠️ Best Practices for Database Management
✅ Let Magic IndexedDB handle database deployment automatically whenever possible.
✅ Use Close()
when you need to release IndexedDB memory but plan to reopen it later.
✅ Only use Delete()
if you are sure you want to wipe all stored data.
✅ If you don’t use migrations, ensure your schema remains static to avoid unintended database resets.